Standalone components are a new feature in Angular 14, but lazyloading standalone components is something you should also use as well!
The lazy loading components feature allows us to easily ‘detach’ components from our build and serve them to our users as per their need, instead of bundling everything and sending it to the user.
How to?
This is actually easier than it sounds. The only requirement is that the lazyloaded component has to be standalone, otherwise this won’t work. So take a look at the app-routing.module.ts
file:
import { NgModule } from '@angular/core';
import { Route, RouterModule, Routes } from '@angular/router';
import { DefaultComponent } from './default/default.component';
const routes: Routes = [
{ path: '', pathMatch: 'full', redirectTo: 'home' },
{ path: 'home', component: DefaultComponent },
{
path: 'standalone',
loadComponent: () =>
import('./standalone/standalone.component').then(
(m) => m.StandaloneComponent
),
},
{
path: 'standalone2',
loadComponent: () =>
import('./standalone2/standalone2.component').then(
(m) => m.StandaloneComponent2
),
},
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule],
})
export class AppRoutingModule {}
As you could see in the code above, we have used a new key in the router object, named loadComponet
`, and imported the component as per request. Using this way, we don’t have to import the components in the module itself and call them when navigating, we are doing this lazily.
If we take a look at our networks tab of the browser inspector, we would be able to see that the components are loading lazily while navigating between routes.
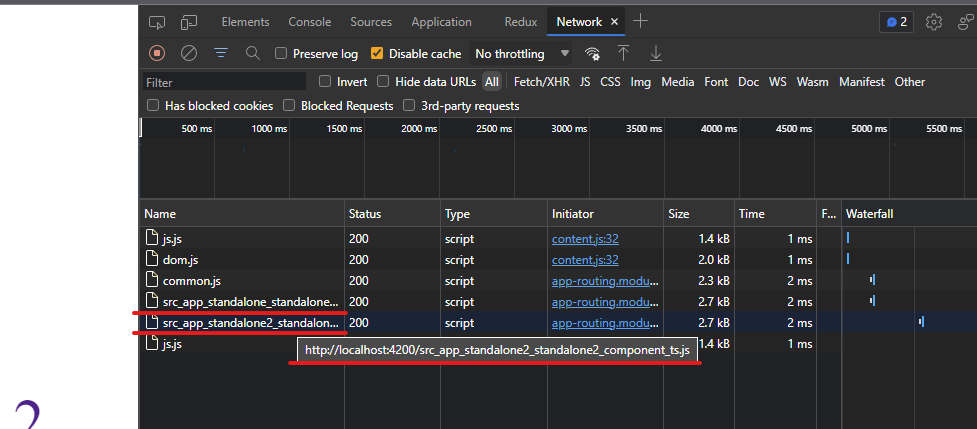
Conclusion
Lazyloading standalone components is a simple-to-implement, yet potent feature for enhancing the performance of Angular applications.
Here is a link to the project’s GitHub page: adnan-halilovic/lazyload-standalone-components-angular: Lazyload Standalone Components in Angular – Youtube Video (github.com)
Thank you for reading the article!
If you like the article and the content, you can follow me on YouTube, Instagram, Linkedin, or any other social networks that you can find listed on my page.